TypeScript
Switching it Up: TypeScript Switch Statements
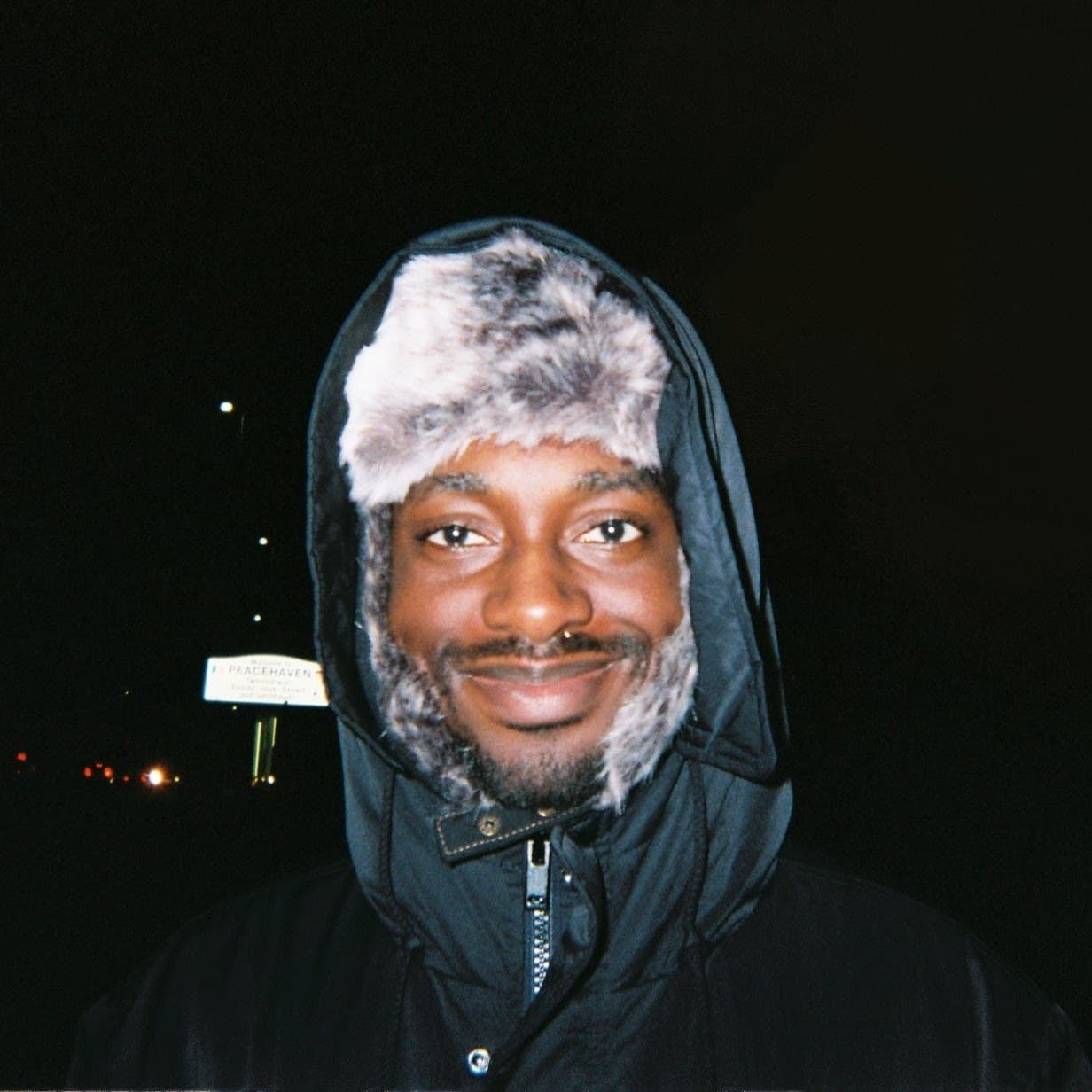
Hey there, TypeScript enthusiasts! If you're diving into the world of TypeScript and wondering how to make your code more expressive and readable, you're in the right place. In this article, we're going to shine a spotlight on one of TypeScript's handy tools: the switch statement.
Introduction.
The switch statement is a conditional statement that matches an expression's value against a series of case clauses. The syntax looks like this:
switch (expression) {
case case1:
code to execute
break;
case case2:
code to execute
break;
default:
statements
}
When a case matches it will execute the code below it, until it reaches a break. We can also have a default case, which will run if none of the other cases do.
Example.
Let's take a look at a straightforward example, we'll create a function that returns the day of the week, given a number:
function getDayOfWeek(dayNumber: number): string | null {
switch (dayNumber) {
case 1:
return "Sunday";
case 2:
return "Monday";
case 3:
return "Tuesday";
case 4:
return "Wednesday";
case 5:
return "Thursday";
case 6:
return "Friday";
case 7:
return "Saturday";
default:
return null;
}
}
This is quite a straightforward switch case, since we're returning inside our switch case we don't even need to include a break. In this code, the getDayOfWeek() function takes a number, "dayNumber" as an argument, and utilizes a switch case to determine the corresponding day of the week. Each case handles a specific day, returning the associated day name. The default case ensures that if an invalid day number is provided, the function returns null.
Switch vs If Statements.
There are a lot of similarities between switch statements and if statements, so you might be wondering when to use each one. In general use whichever you prefer, but in general use a switch statement if you need to check if one value is equal to some other specific values, and use an if statement for more complex conditionals.
Taking our "getDayOfWeek()", we could easily have written this as a series of if statements:
function getDayOfWeek(dayNumber: number) {
if (dayNumber === 1) {
return "Sunday";
}
if (dayNumber === 2) {
return "Monday";
}
if (dayNumber === 3) {
return "Tuesday";
}
if (dayNumber === 4) {
return "Wednesday";
}
if (dayNumber === 5) {
return "Thursday";
}
if (dayNumber === 6) {
return "Friday";
}
if (dayNumber === 7) {
return "Saturday";
}
return null;
}
Switch statements are helpful here as they allow us to avoid repeating our conditional statement.
Break and Fall-through.
A great feature of switch cases is that they will "fall through" until they reach a "break;". This means that if you have one piece of code you want to be executed for multiple switch cases, you can do so by stacking the cases on top of each other.
const input: string = 'apple';
let result = null;
switch (input) {
case 'apple':
case 'banana':
case 'orange':
result = 'fruit';
break;
case 'carrot':
case 'onion':
result = 'vegetable';
break;
default:
result = null;
break;
}
console.log(result); //fruit
You can still execute code in the blocks without a break:
function createUser(type: 'admin' | 'user' | 'guest') {
const user: { name: string; permissions: string[] } = {
name: 'John Doe',
permissions: [],
};
switch (type) {
case 'guest':
user.permissions.push('read');
case 'user':
user.permissions.push('write');
case 'admin':
user.permissions.push('delete');
break;
}
}
In this case, we have some kind of user system - guests can only read content, users can read and write, and admins can read, write and delete. With switch statements falling through we can combine this logic into one simple switch statement, without having to repeat code.
The TypeScript Part.
All of the code so far has been fairly plain JavaScript, so you might be wondering what switch statements mean for TypeScript. It has some implications for type narrowing. If we take a look at our previous "getDayOfWeek()" function, the return type I've provided is "string | null". If we take this away and let TypeScript infer the return type, here's what we get for the signature of the function:
function getDayOfWeek(dayNumber: number): "Sunday" | "Monday" | "Tuesday" | "Wednesday" | "Thursday" | "Friday" | "Saturday" | null
If you take a look at our function, the only values it can return are one of the days of the week, or null. TypeScript is also able to see this, and can infer the exact type for the function.
Conclusion.
And there you have it – the magical world of switch cases in TypeScript unveiled! We've journeyed through the basics, explored advanced techniques, and witnessed firsthand how this nifty tool can transform your code. Hopefully, with this article, you were able to get a good understanding of the switch statement in TypeScript, how to handle switch cases, and when to use the statement. If you liked this article, feel free to share it!
read more.
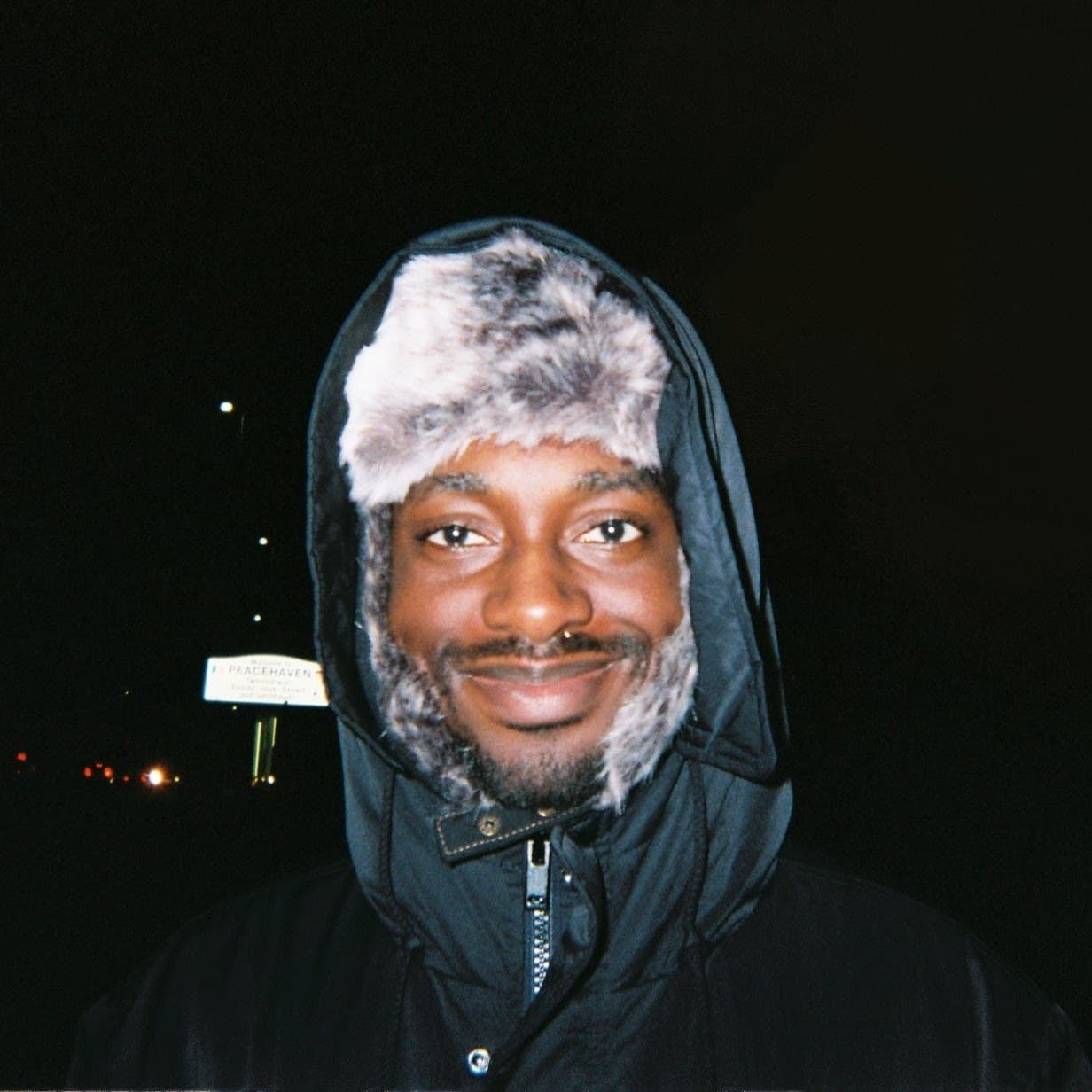
I'm a full-stack developer from the UK. I'm currently looking for graduate and freelance software engineering roles, so if you liked this article, feel free to reach out.