JavaScript
How to Conditionally Add a Property to an Object or Array in JavaScript
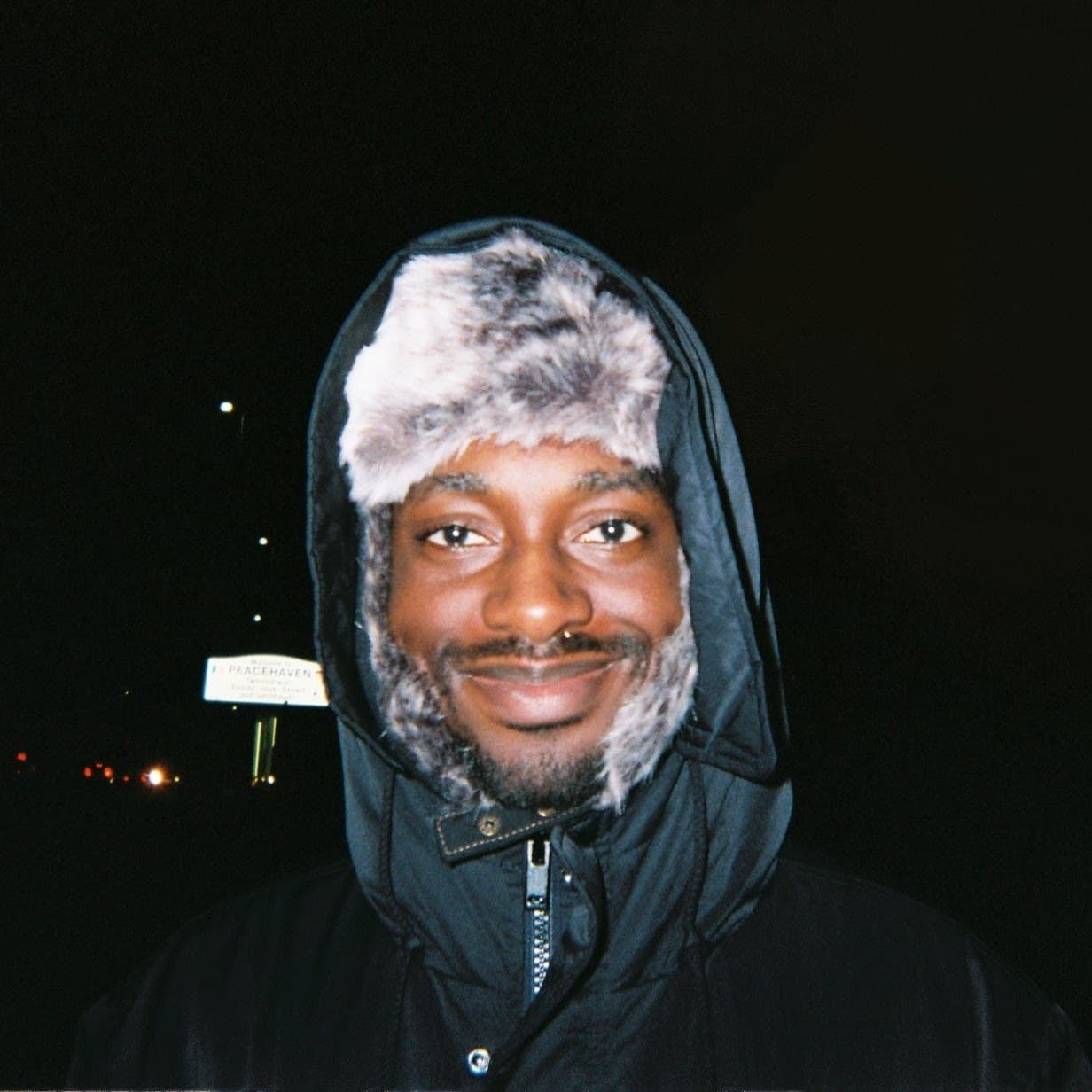
JavaScript objects and arrays are very dynamic and flexible, and one problem you're likely to run into is how to conditionally add properties to your objects/arrays. The short version is there's no native way to conditionally add a property to an object or array literal, but there are some handy shortcuts.
The Obvious Way.
If your object/array is mutable, then of course you can just conditionally call the code that adds the key:
let myObject = {
foo: 'foo',
};
if (condition) myObject.bar = 'bar';
//Or an array
let myArray = ['foo'];
if (condition) myArray.push('bar');
A Handy Shortcut.
You might not like the first option for several reasons. Personally, as a React developer, I'm a big fan of immutable variables, and as such I prefer to keep my variables as consts whenever I can. As such, it would be nice if I could add the property conditionally during initialisation, and thankfully there is:
let myObject = {
foo: 'foo',
...(condition && { bar: 'bar' }),
};
// Or an array
let myArray = ['foo', ...(condition ? ['bar'] : [])];
This looks a little weird, so let's break it down.
Objects.
Firstly we have the spread syntax. The spread syntax, (or spread operator, as you might see people refer to it as) can be used to expand an iterable, or expand object properties. You can think of it as turning a collection of things into individual things. If you spread an object inside an object literal, those properties get added to that object, and if you spread an iterable inside an array literal, the items get added to that array.
So the first part of this is that we're adding whatever is inside our brackets to our object/array.
Inside our brackets, for an object, we have the logical AND operator. This operator is lazy. if the first value is falsy, then the whole operator short-circuits to the first operator, since there's no way the whole thing can be true. If it's true, then it just returns the second value, since that's the only thing that affects whether the whole thing is true.
"False and X" is always false, and likewise, "True and X" is just the same as True.
Inside our conditional, if the condition is false we don't get an object, but thankfully that falsy value is just ignored. If the condition is true we get the second value which is an object, which gets spread and added to our object.
Arrays.
For an array the logic is exactly the same, but JavaScript won't let you spread something into an array that isn't iterable. Instead of just returning false with the logical AND operator, we can use the ternary operator to just return an empty array, which then gets spread, but nothing gets added to our array.
You could also use a conditional for an object:
let myObject = {
foo: 'foo',
...(condition ? { bar: 'bar' } : {}),
};
But the AND operator is a little shorter.
Conclusion.
So to sum everything up, if you want to add a property conditionally to an object/array in JavaScript there are two ways. If you don't mind your object/array being mutable then you can just set a property on the object/array conditionally. Otherwise you can use this handy shortcut:
let myObject = {
foo: 'foo',
...(condition && { bar: 'bar' }),
};
// Or an array
let myArray = ['foo', ...(condition ? ['bar'] : [])];
Thanks for reading! I hope you liked this article - feel free to share it, or help me out with any feedback.
read more.
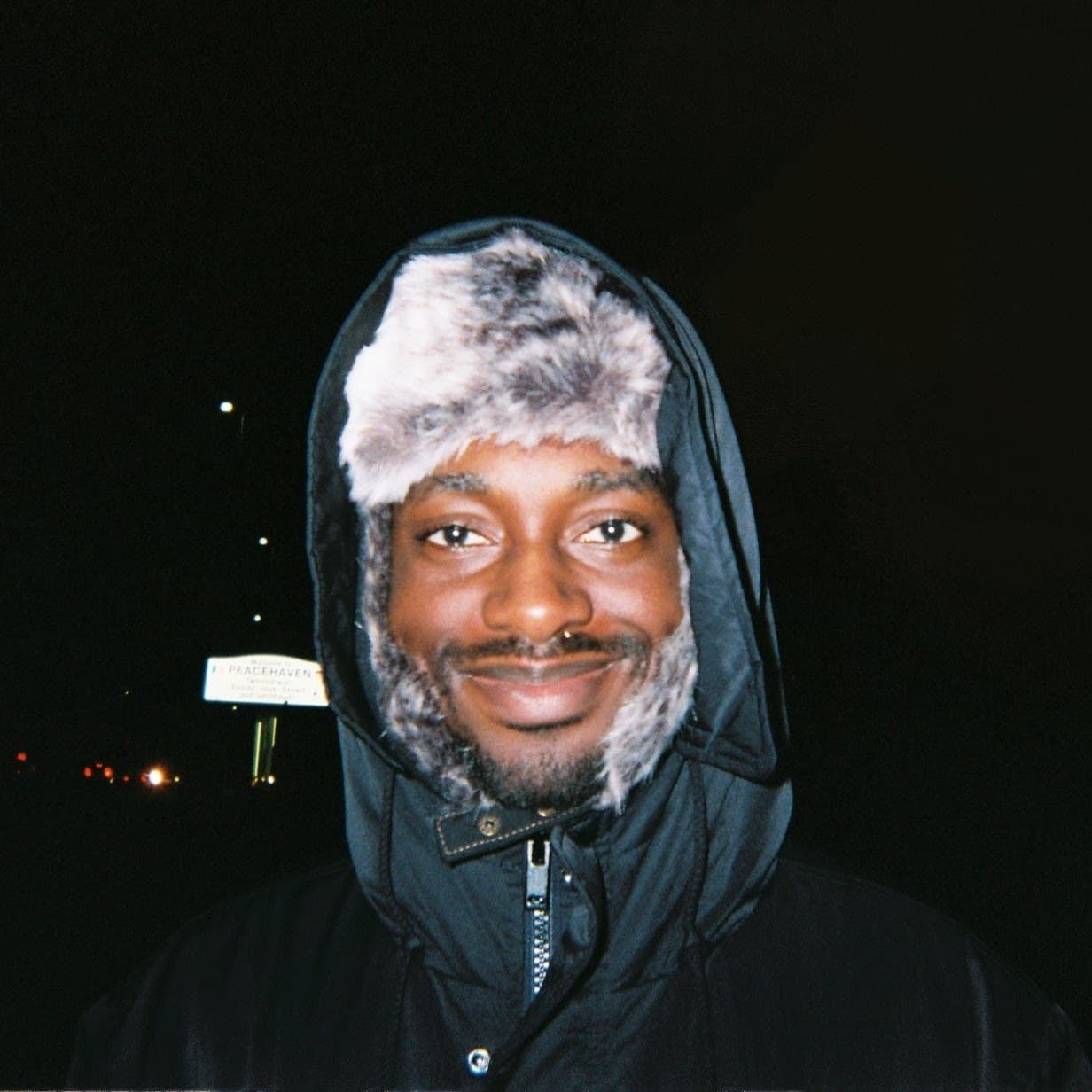
I'm a full-stack developer from the UK. I'm currently looking for graduate and freelance software engineering roles, so if you liked this article, feel free to reach out.